Quick Start Guide#
Installation#
Data Morph can be installed from PyPI using pip
:
$ python -m pip install data-morph-ai
Alternatively, Data Morph can be installed with conda
by specifying the conda-forge
channel:
$ conda install -c conda-forge data-morph-ai
Usage#
Once installed, Data Morph can be used on the command line or as an importable Python package.
Command line usage#
Run data-morph
on the command line:
$ data-morph --start-shape panda --target-shape star
This produces the following animation in the newly-created morphed_data
directory
within your current working directory:
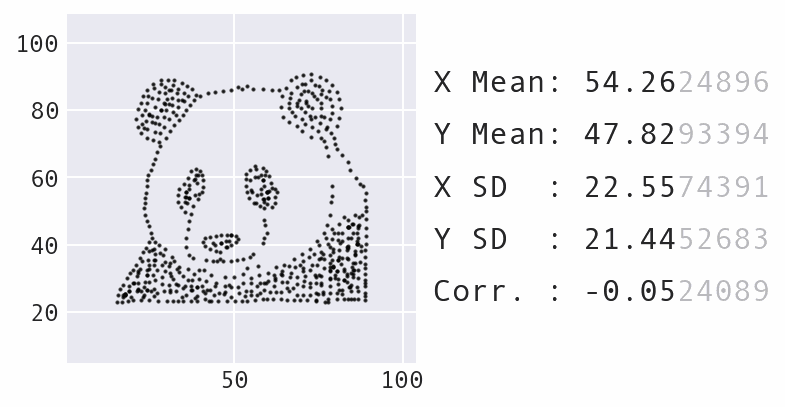
You can smooth the transition with the --ease
or --ease-in
and --ease-out
flags.
The --freeze
flag allows you to start the animation with the specified number of frames
of the initial shape:
$ data-morph --start-shape panda --target-shape star --freeze 50 --ease
Here is the resulting animation:
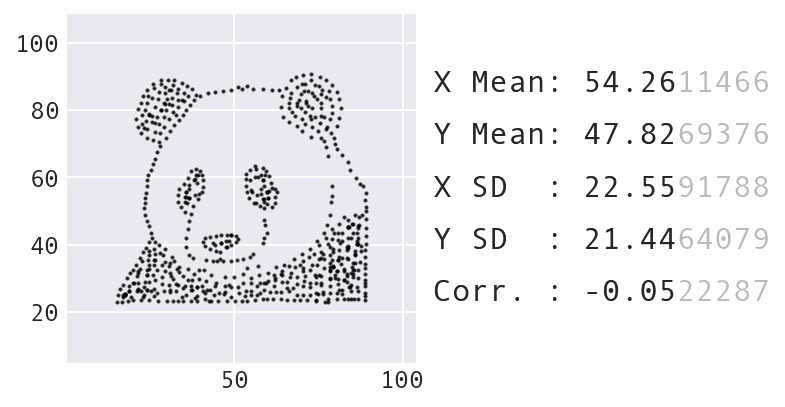
Morphing the panda Dataset
into the star Shape
with easing.#
The CLI generates animations for the Cartesian product of the datasets and shapes provided. For example, if you wanted to morph both the music and soccer datasets into both the heart and diamond shapes (i.e., four animations), you could run the command below:
$ data-morph --start-shape music soccer --target-shape heart diamond
Tip
When doing generating multiple animations, it is recommended that you also specify
the number of jobs you want to run in parallel (limited by the number of CPU cores
on your machine). If you pass 0
, Data Morph will run as many as possible:
$ data-morph --start-shape music soccer --target-shape heart diamond --workers 0
If you have the GNU parallel
command on your machine, you can use it to run
a slightly faster parallelized Data Morph (since it incurs less Python overhead),
in which case you don’t need to provide the worker count:
$ parallel --progress -j0 \
> data-morph --start-shape {1} --target-shape {2} \
> ::: music soccer ::: heart diamond
Check out the GNU parallel documentation for more options.
See all available CLI options by passing in --help
or consulting the CLI Reference:
$ data-morph --help
Python usage#
The DataMorpher
class performs the morphing from a Dataset
to a Shape
.
Any DataFrame
with numeric columns x
and y
can be a Dataset
.
Use the DataLoader
to create the Dataset
from a file or use a built-in dataset:
from data_morph.data.loader import DataLoader
dataset = DataLoader.load_dataset('panda')
For morphing purposes, all target shapes are placed/sized based on aspects of the Dataset
.
All shapes are accessible via the ShapeFactory
:
from data_morph.shapes.factory import ShapeFactory
shape_factory = ShapeFactory(dataset)
target_shape = shape_factory.generate_shape('star')
With the Dataset
and Shape
created, here is a minimal example of morphing:
from data_morph.morpher import DataMorpher
morpher = DataMorpher(
decimals=2,
in_notebook=False, # whether you are running in a Jupyter Notebook
output_dir='data_morph/output',
)
result = morpher.morph(
start_shape=dataset,
target_shape=target_shape,
freeze_for=50,
ease_in=True,
ease_out=True,
)
Note
The result
variable in the above code block is a DataFrame
of the data
after completing the specified iterations of the simulated annealing process. The DataMorpher.morph()
method is also saving plots to visualize the output periodically and make an animation; these end up in
data_morph/output
, which we set as DataMorpher.output_dir
.
In this example, we morphed the built-in panda Dataset
into the star Shape
. Be sure to try
out the other built-in options:
The
DataLoader.AVAILABLE_DATASETS
attribute contains a list of available datasets, which are also visualized in theDataLoader
documentation.The
ShapeFactory.AVAILABLE_SHAPES
attribute contains a list of available shapes, which are also visualized in theShapeFactory
documentation.
For further customization, the Custom Datasets tutorial discusses how to generate custom input datasets, and the Shape Creation tutorial discusses how to generate custom target shapes.